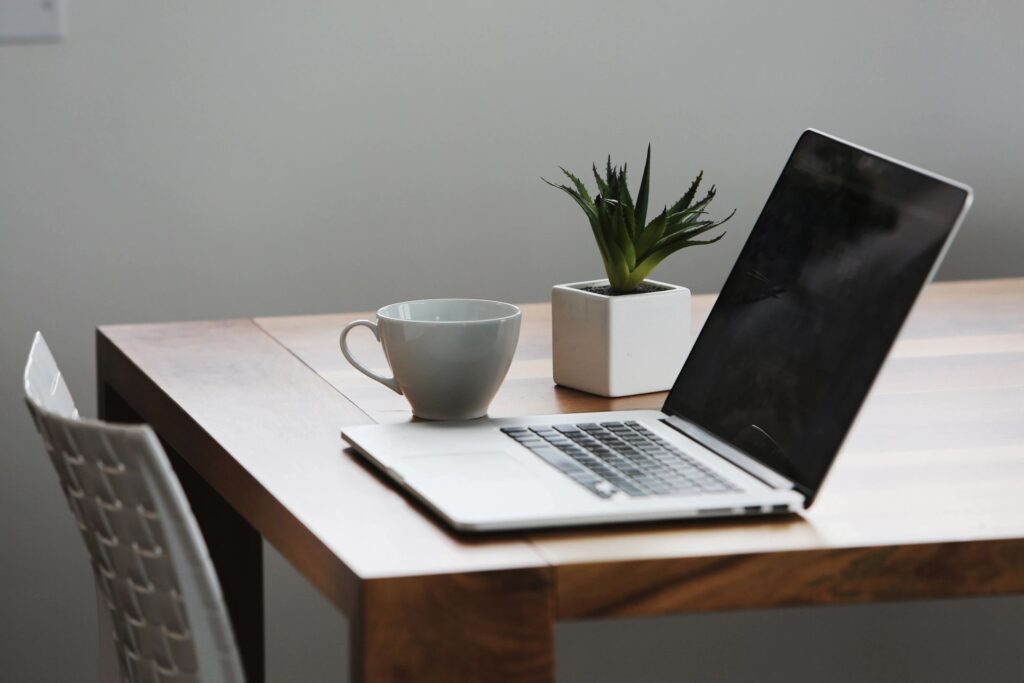
An outline for children to learn Python in an effortless manner
This outline provides a comprehensive guide for children to learn Python in an easy and interactive way.
- Introduction to Python
- Setting up the Environment
- Understanding Variables and Data Types
- Conditional Statements
- Loops
- Functions
- Arrays and Lists:
- Working with Files
- Object-Oriented Programming
- Project-Based Learning
Introduction to Python: To start, children will be introduced to the basics of Python, including its history and its real-world applications.
Setting up the Environment: Children will learn how to set up their programming environment, including downloading and installing Python and a text editor.
Understanding Variables and Data Types: Children will learn about variables, which store data, and data types, such as strings, numbers, and booleans. They will also practice using variables and data types in operations.
# Assign a string value to a variable
name = "John"
# Assign a numeric value to a variable
age = 10
# Print the value of the variables
print("My name is", name)
print("My age is", age)
Conditional Statements: Children will learn about conditional statements, such as ‘if-else’ statements, which allow the program to make decisions based on specific conditions.
# Check if a number is positive or negative
num = 10
if num > 0:
print("Positive")
else:
print("Negative")
Loops: Loops are a key programming concept and children will learn how to use ‘for’ and ‘while’ loops to repeat actions
# Print numbers from 1 to 10 using a for loop
for i in range(1, 11):
print(i)
# Print numbers from 1 to 10 using a while loop
i = 1
while i <= 10:
print(i)
i += 1
Functions: Functions are blocks of code that can be reused. Children will learn how to define and call functions in Python.
# Define a function to calculate the square of a number
def square(num):
result = num * num
return result
# Call the function
num = 10
result = square(num)
print("The square of", num, "is", result)
Arrays and Lists: Children will learn about arrays and lists, data structures used to store multiple values in a single variable
# Create a list of numbers
numbers = [1, 2, 3, 4, 5]
# Print the elements of the list
for number in numbers:
print(number)
Working with Files: Children will learn how to read from and write to files in Python, a useful skill when working with data
# Write to a file
with open("file.txt", "w") as file:
file.write("Hello, World!")
# Read from a file
with open("file.txt", "r") as file:
content = file.read()
print(content)
Object-Oriented Programming: Children will be introduced to Object-Oriented Programming, a way of organizing code and data into reusable units called objects
Project-Based Learning: Finally, children will apply their new skills by completing a fun and interactive project.
By following this outline, children will have a strong foundation in Python programming and be able to create their own projects with
Here are some additional resources that children can use to continue learning Python and improve their skills:
- Codecademy’s Learn Python Course: This interactive course covers the basics of Python and provides hands-on projects to reinforce the concepts.
- Python.org’s Beginner’s Guide: This guide provides a comprehensive introduction to Python and its syntax, with a focus on beginners.
- Pygame: Pygame is a library for making games in Python. It’s a fun way for children to apply their Python skills to create games and interactive projects.
- Google’s Python Class: Google offers a free Python class for people with a little bit of programming experience who want to learn Python. The class includes written materials, lecture videos, and exercises to practice.
- Project Euler: Project Euler is a website that provides challenging math problems that can be solved using programming. Children can use Python to solve these problems and strengthen their skills.
- Python for Data Science Handbook: This book provides an introduction to Python for data science, including topics such as NumPy, Pandas, and Matplotlib. It’s a great resource for children who are interested in learning about data science.
- YouTube Tutorials: There are many YouTube tutorials on Python programming that can help children continue their learning. Some popular channels include Corey Schafer, FreeCodeCamp, and Traversy Media.
By using these resources, children can continue to improve their Python skills and explore new topics in more depth.
.