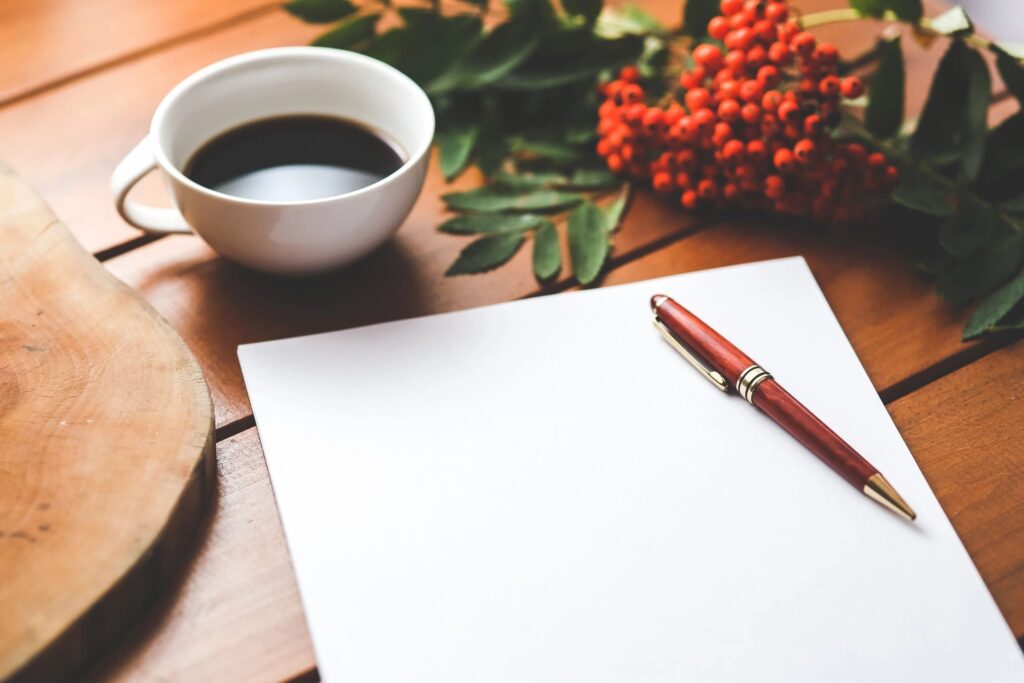
Big O notation with examples
Response from ChatGPT
Introduction
In computer science, we often need to compare the performance of algorithms or functions. Big O notation is a way to describe the performance of an algorithm in terms of the size of its input. It provides an upper bound on the growth rate of the function, representing how quickly the function’s runtime increases as the size of the input increases.
Definition
Big O notation describes the upper bound of the growth rate of a function. It is written as O(f(n)), where f(n) is a function that represents the growth rate of the algorithm, and n represents the size of the input.
Examples
Let’s look at some common examples of Big O notation:
- O(1): A constant time algorithm. It takes the same amount of time to complete, regardless of the size of the input. Examples include accessing a single element in an array or performing a basic arithmetic operation.
- O(log n): A logarithmic time algorithm. The time complexity increases logarithmically with the size of the input. Examples include binary search, where the search space is halved with each comparison.
- O(n): A linear time algorithm. The time complexity increases linearly with the size of the input. Examples include iterating through an array to find a specific value.
- O(n log n): A quasilinear time algorithm. The time complexity increases slightly faster than linearly with the size of the input. Examples include sorting algorithms like Merge Sort and Quick Sort.
- O(n^2): A quadratic time algorithm. The time complexity increases exponentially with the size of the input. Examples include nested loops iterating through an array.
- O(2^n): An exponential time algorithm. The time complexity doubles with each additional input. Examples include the brute force approach to the Traveling Salesman Problem.
- O(n!): A factorial time algorithm. The time complexity grows factorially with the size of the input. Examples include the brute force approach to the Traveling Salesman Problem with no pruning.
Conclusion
Big O notation is a powerful tool for understanding the performance of algorithms. By comparing the upper bounds of growth rates for different algorithms, we can choose the most efficient one for a given problem. Remember that Big O notation only provides an upper bound on growth rate, so it’s important to consider other factors like space complexity and constant factors when comparing algorithms.
References: