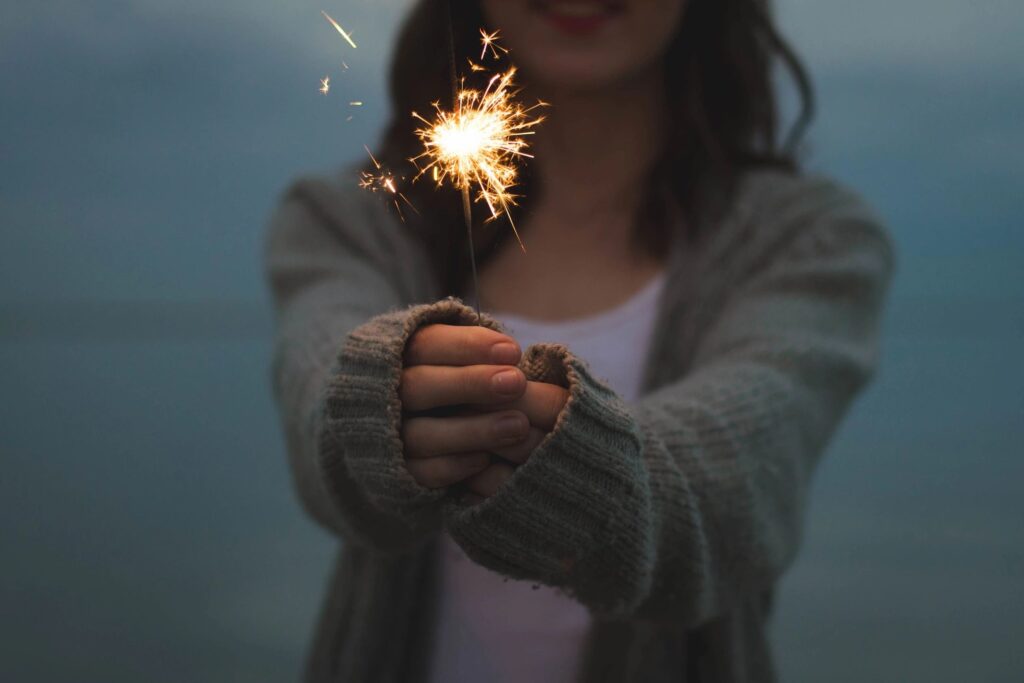
How to resolve the error 'ChatGPT is at capacity right now
The error message ‘ChatGPT is at capacity right now’ is encountered when the demand for the ChatGPT API exceeds its capacity to process requests. This is a temporary issue, and there are a few steps you can take to resolve it.
1.Wait and Retry:
One option is to simply wait a few minutes and then retry your request. During periods of high demand, the API may become temporarily unavailable, but it should become accessible again shortly.
2. Use Off-Peak Hours:
Another option is to use the API during off-peak hours, when the demand is lower. This may help ensure that your requests are processed more quickly and reliably.
3. Implement Retry Logic:
If you are using the API programmatically, it is a best practice to implement retry logic in your code. This involves retrying the request after a set amount of time if the API returns an error. For example, you could wait for five seconds before retrying the request, and increase the wait time after each subsequent failure.
# Example retry logic in Python
import time
def make_request():
# code to make API request
response = ...
return response
# Maximum number of retries
MAX_RETRIES = 5
# Wait time between retries (in seconds)
WAIT_TIME = 5
# Counter to keep track of the number of retries
retry_count = 0
# Make the API request
response = make_request()
# Check if the API returned an error
while response is None and retry_count < MAX_RETRIES:
# Wait for WAIT_TIME seconds before retrying
time.sleep(WAIT_TIME)
# Increment the retry count
retry_count += 1
# Make the API request again
response = make_request()
# Handle the response
if response is not None:
# Use the response
...
else:
# Maximum number of retries reached
...
4 . Increase Request Batching:
If you are making multiple requests to the API in a short period of time, consider increasing the batch size of your requests. This can reduce the number of requests being made and help the API process your requests more efficiently.
# Example of request batching in Python
import requests
# List of texts to be processed by the API
texts = [
"Hello, how are you today?",
"What's the weather like in Seattle?",
"What's the latest news?"
]
# Batch size
BATCH_SIZE = 2
# Number of batches
num_batches = len(texts) // BATCH_SIZE + (len(texts) % BATCH_SIZE != 0)
# Split the texts into batches
batches = [texts[i:i + BATCH_SIZE] for i in range(0, len(texts), BATCH_SIZE)]
# Process each batch
for batch in batches:
# Code to format the API request
data = ...
headers = ...
# Make the API request
response = requests.post(url, data=data, headers=headers)
# Handle the response
if response.status_code == 200:
# Use the response
...
else:
# Handle the error
...
5. Utilize Queuing:
If you have a large number of requests to process, consider utilizing a queueing system to manage the requests. The queueing system can help ensure that requests are processed in a predictable order and that the API is not overwhelmed with requests.
# Example of utilizing a queueing system in Python
import queue
import threading
# Queue to hold the requests
q = queue.Queue()
# Number of worker threads
NUM_WORKER_THREADS = 2
# Function to process a request
def process_request(q):
while True:
# Get the next request from the queue
request = q.get()
# Make the API request
response = ...
# Handle the response
if response is not None:
# Use the response
...
else:
# Handle the error
...
# Signal that the task is done
q.task_done()
# Start the worker threads
for i in range(NUM_WORKER_THREADS):
t = threading.Thread(target=process_request, args=(q,))
t.daemon = True
t.start()
# Add the requests to the queue
for request in requests:
q.put(request)
# Wait for all requests to be processed
q.join()
These are just a few examples to help you resolve the “ChatGPT is at capacity right now” error. By taking these steps, you can help ensure that your requests are processed smoothly and efficiently, even during periods of high demand.