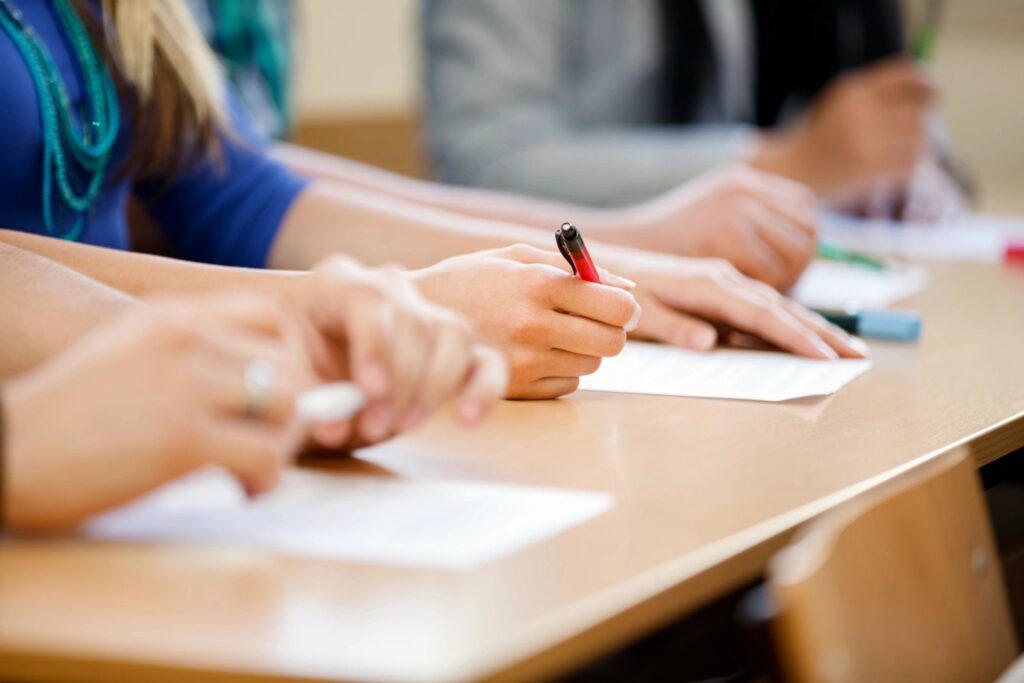
Remove k-th last element from linked list
Question:
Given a singly linked list and an integer k, remove the kth last node from the linked list and return the new linked list. Implement the solution using a single pass and constant space.
Example:
Input: 1 -> 2 -> 3 -> 4 -> 5, k = 2 Output: 1 -> 2 -> 3 -> 5
Explanation: In the input linked list, the 2nd last node is 4. After removing it, the new linked list becomes 1 -> 2 -> 3 -> 5.
Answer from ChatGPT
To remove the k-th last element from a singly linked list in a single pass, we can use two pointers. The first pointer starts at the beginning of the list, and the second pointer starts k steps ahead of the first pointer. Then, we move both pointers simultaneously until the second pointer reaches the end of the list. At this point, the first pointer will be pointing to the k-th last element.
To remove the k-th last element, we simply update the next pointer of the (k-1)-th element to skip over the k-th last element. However, to handle the edge case where we need to remove the first element of the list, we need to maintain a “dummy” node at the beginning of the list.
Here is the code that implements this approach:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def remove_kth_last(head: ListNode, k: int) -> ListNode:
dummy = ListNode(0, head)
first = second = dummy
# Move the second pointer k steps ahead of the first pointer
for i in range(k):
second = second.next
# Move both pointers simultaneously until the second pointer reaches the end of the list
while second.next:
first = first.next
second = second.next
# Remove the k-th last element by updating the next pointer of the (k-1)-th element
first.next = first.next.next
return dummy.next
The time complexity of this approach is O(n), where n is the length of the linked list, since we traverse the list once. The space complexity is O(1), since we only use constant extra space to maintain the two pointers and the dummy node.
Explain why using a dummy node is beneficial, and provide some examples of situations in which a dummy node can be used
A dummy node is a “dummy” or “sentinel” node that serves as a placeholder at the beginning of a linked list or other data structure. It does not contain any meaningful data, but it simplifies the implementation of certain algorithms by providing a fixed point of reference.
In the context of the problem of removing the k-th last element from a linked list, using a dummy node allows us to handle the edge case where we need to remove the first element of the list. Without a dummy node, we would need to check whether we are removing the first element of the list, and handle this case separately. By using a dummy node, we can treat all elements of the list uniformly, without having to write special case handling code.
There are several benefits to using a dummy node in certain algorithms. Some of these benefits include:
- Simplified edge case handling: As explained above, using a dummy node can simplify the handling of edge cases in algorithms.
- Better readability: By providing a fixed point of reference, a dummy node can make the code easier to read and understand, since it makes the algorithm’s logic more explicit.
- Improved performance: In some cases, using a dummy node can improve the performance of an algorithm by reducing the number of special cases that need to be handled.
There are several situations where using a dummy node can be beneficial, such as:
- Linked list traversal: When traversing a linked list, a dummy node can be used as a placeholder at the beginning of the list, simplifying the implementation of certain algorithms.
- Sorting algorithms: In some sorting algorithms, a dummy node can be used to simplify the handling of edge cases, such as when sorting an empty list or a list with only one element.
- Binary tree traversal: In binary tree traversal algorithms, a dummy node can be used as a placeholder for the root node, simplifying the implementation of certain traversal algorithms.
Overall, using a dummy node can simplify the implementation of certain algorithms, and can lead to better performance and readability in some cases.
Please provide examples of questions and solutions that illustrate each key point you mentioned in your previous answer regarding the benefits of using a dummy node.
here are some additional examples of questions and solutions that use a dummy node:
- Simplified edge case handling
Question: Given a sorted array of integers, write a function to remove all duplicates from the array and return the resulting array.
Solution: We can use a two-pointer approach to iterate over the array and remove duplicates. To simplify the edge case handling when the array is empty or contains only one element, we can prepend a dummy value at the beginning of the array. This way, we can treat all elements of the array uniformly, without having to write special case handling code. Here is the code:
def remove_duplicates(arr):
arr = [float('-inf')] + arr
slow, fast = 1, 1
while fast < len(arr):
if arr[fast] != arr[fast - 1]:
arr[slow] = arr[fast]
slow += 1
fast += 1
return arr[1:slow]
- Better readability
Question: Given a linked list of integers, write a function to reverse the list in place and return the new head node.
Solution: We can use a recursive algorithm to reverse the linked list. To make the code more readable, we can use a dummy node as a placeholder for the new head node. Here is the code:
def reverse_list(head):
dummy = ListNode(0)
_reverse_list(head, dummy)
return dummy.next
def _reverse_list(node, dummy):
if not node:
return
_reverse_list(node.next, dummy)
dummy.next = node
dummy = dummy.next
- Improved performance
Question: Given a linked list of integers, write a function to remove all nodes with odd values and return the new head node.
Solution: We can use a two-pointer approach to iterate over the linked list and remove nodes with odd values. To improve the performance of the algorithm, we can use a dummy node as a placeholder for the new head node. This way, we can avoid having to write special case handling code for the head node. Here is the code:
def remove_odd_nodes(head):
dummy = ListNode(0, head)
prev, curr = dummy, head
while curr:
if curr.val % 2 == 1:
prev.next = curr.next
else:
prev = curr
curr = curr.next
return dummy.next
Overall, using a dummy node can simplify edge case handling, make the code more readable, and improve performance in some cases.