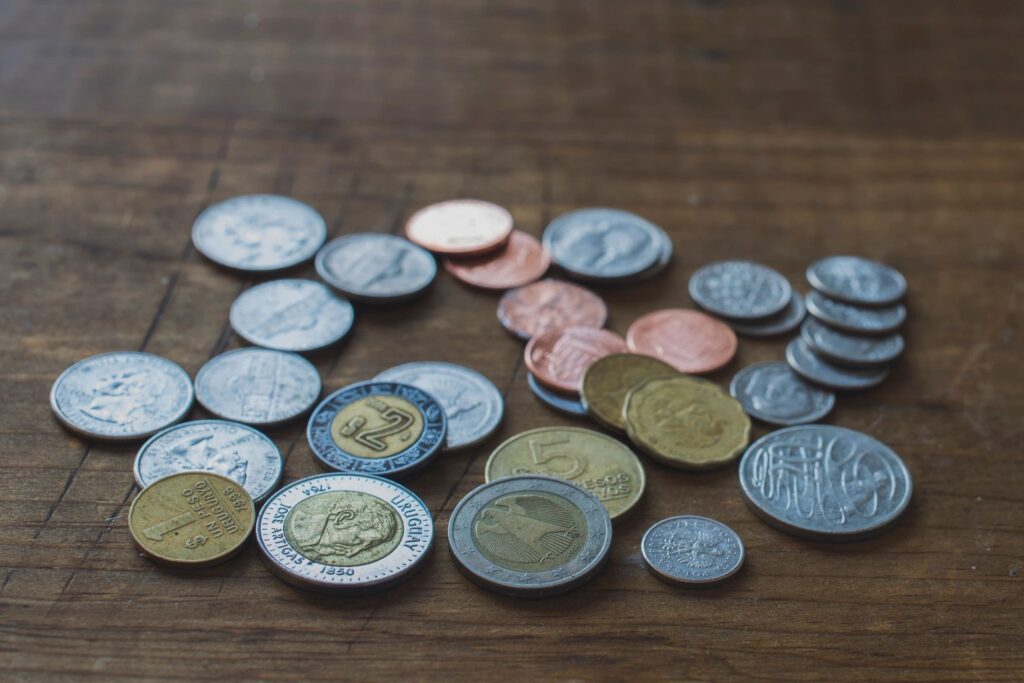
coin amount
Q:
Given a list of possible coins in cents, and an amount (in cents) n, return the minimum number of coins needed to create the amount n. If it is not possible to create the amount using the given coin denomination, return None. Here’s an example and some starter code: def make_change(coins, n):
A:
def make_change(coins, n):
memo = {} # Memoization dictionary to store results for subproblems
def min_coins(amount):
if amount in memo:
return memo[amount]
if amount == 0:
return 0
min_coins_needed = float('inf') # Initialize with a large value
for coin in coins:
if amount - coin >= 0:
# Recursively calculate the number of coins needed for the remaining amount
coins_needed = min_coins(amount - coin) + 1
# Update the minimum coins needed if the current option requires fewer coins
min_coins_needed = min(min_coins_needed, coins_needed)
# Store the result for the current amount in the memoization dictionary
memo[amount] = min_coins_needed
return min_coins_needed
result = min_coins(n)
return result if result != float('inf') else None
# Test example
print(make_change([1, 5, 10, 25], 36)) # Output: 3 (as 36 = 25 + 10 + 1)
The explanation and provide line-by-line documentation for the code:
def make_change(coins, n):
memo = {} # Memoization dictionary to store results for subproblems
Here, we define the make_change function that takes two parameters: coins, which is a list of possible coin denominations, and n, which is the target amount we want to create using the coins. We initialize an empty dictionary memo, which will be used to store the results of subproblems to avoid redundant calculations and improve efficiency.
def min_coins(amount):
if amount in memo:
return memo[amount]
We define an inner function min_coins, which is a recursive function to calculate the minimum number of coins needed to make the given amount. Before performing any calculations, we check if the amount is already in the memo dictionary. If it is, that means we have previously calculated and stored the result for this amount, so we directly return it to avoid re-computation.
if amount == 0:
return 0
The base case of the recursion: If the amount is 0, it means we don't need any coins, so we return 0.
min_coins_needed = float('inf') # Initialize with a large value
We initialize the variable min_coins_needed to a very large value (float('inf')). This value will be used to store the minimum number of coins needed to create the current amount, and we will update it to a smaller value as we find better solutions.
for coin in coins:
if amount - coin >= 0:
# Recursively calculate the number of coins needed for the remaining amount
coins_needed = min_coins(amount - coin) + 1
# Update the minimum coins needed if the current option requires fewer coins
min_coins_needed = min(min_coins_needed, coins_needed)
We iterate through each coin denomination in the coins list. For each coin, we check if using this coin reduces the current amount (i.e., amount - coin >= 0). If it does, we recursively calculate the number of coins needed to create the remaining amount after deducting the current coin (amount - coin), and we add 1 to the result to account for using the current coin.
We then compare the coins_needed (number of coins needed with the current coin) with the current min_coins_needed. If coins_needed is smaller, it means using the current coin results in fewer coins needed, so we update min_coins_needed with this value.
# Store the result for the current amount in the memoization dictionary
memo[amount] = min_coins_needed
After iterating through all possible coin denominations and calculating the minimum coins needed for the current amount, we store this result in the memo dictionary. This way, if we encounter the same amount again in the future, we can directly use the pre-calculated result from the dictionary instead of recalculating it.
return min_coins_needed
Finally, we return the minimum number of coins needed to create the given amount.
result = min_coins(n)
return result if result != float('inf') else None
In the make_change function, we call the min_coins function with the input n (the target amount). The result is stored in the result variable. If result is not equal to float('inf'), it means we were able to create the target amount using the given coin denominations, so we return the result. Otherwise, we return None to indicate that it is not possible to create the amount using the given coins.
print(make_change([1, 5, 10, 25], 36)) # Output: 3 (as 36 = 25 + 10 + 1)
In this test example, we call the make_change function with the coins list containing denominations [1, 5, 10, 25] and the target amount 36. The output will be 3, as it requires a minimum of 3 coins (25 cents, 10 cents, and 1 cent) to make 36 cents.