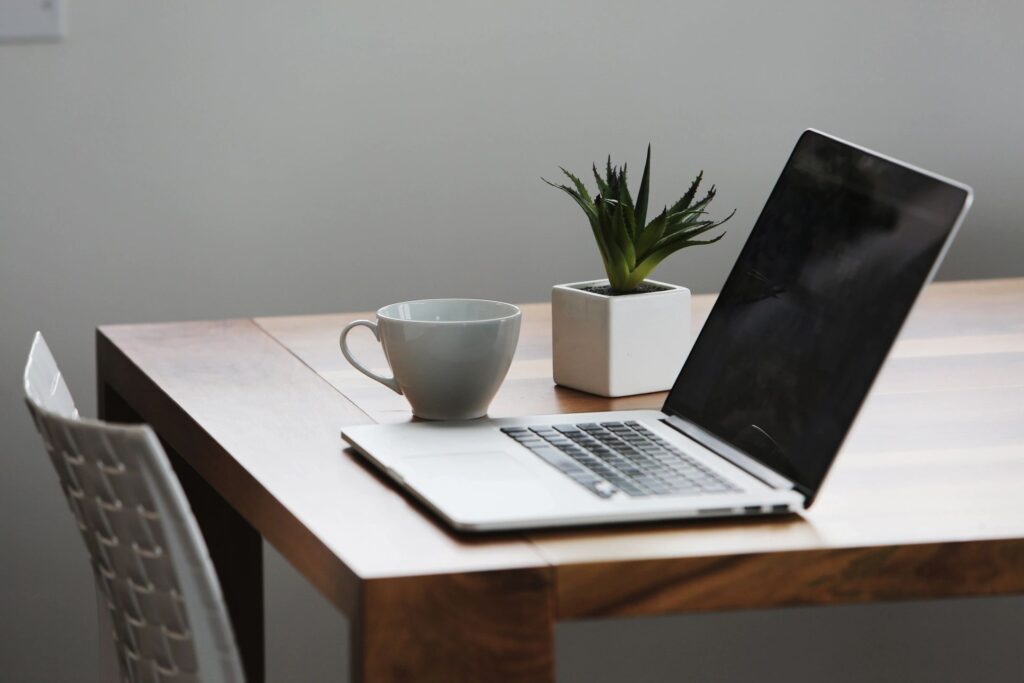
Find the single number in an array of integers
Question:
Given an array of integers, arr, where all numbers occur twice except one number which occurs once, find the number. Your solution should ideally be O(n) time and use constant extra space. Example: Input: arr = [7, 3, 5, 5, 4, 3, 4, 8, 8] Output: 7
Response from ChatGPT
class Solution(object):
def findSingle(self, nums):
# initialize the result variable to 0
res = 0
# iterate over each number in the array
for num in nums:
# perform bitwise XOR operation with the current number
res ^= num
# return the result variable, which will contain the single number
return res
# example usage
nums = [7, 3, 5, 5, 4, 3, 4, 8, 8]
print(Solution().findSingle(nums)) # prints 7
Here’s an explanation of how the algorithm works:
- We initialize a variable called
res
to 0. This variable will be used to store the result of performing the bitwise XOR operation on all the numbers in the array. - We iterate over each number in the array, and for each number, we perform the bitwise XOR operation with the current value of
res
. This has the effect of cancelling out all the numbers that occur twice, leaving only the single number that occurs once. - Finally, we return the value of
res
, which should contain the single number that occurs once.
This solution has a time complexity of O(n) and a space complexity of O(1), since it uses only a single variable to store the result.
问题:给定一个整数数组arr,其中所有数字都出现两次,除了一个数字只出现一次,找到这个数字。你的解决方案应该尽可能地在O(n)的时间复杂度内运行,并使用恒定的额外空间。
示例:
输入:arr = [7, 3, 5, 5, 4, 3, 4, 8, 8]
输出:7
class Solution(object):
def findSingle(self, nums):
# 将结果变量初始化为0
res = 0
# 遍历数组中的每个数字
for num in nums:
# 对当前数字执行位异或运算
res ^= num
# 返回结果变量,其中包含出现一次的数字
return res
# 示例用法
nums = [7, 3, 5, 5, 4, 3, 4, 8, 8]
print(Solution().findSingle(nums)) # 输出 7
以下是算法的工作原理:
我们将一个名为 res 的变量初始化为 0。此变量将用于存储对数组中所有数字执行位异或运算的结果。
我们遍历数组中的每个数字,并对每个数字执行位异或运算,使用当前 res 变量的值。这样就能消除所有出现两次的数字,只剩下出现一次的数字。
最后,我们返回 res 变量的值,其中应包含出现一次的数字。
该解决方案的时间复杂度为 O(n),空间复杂度为 O(1),因为它只使用了一个变量来存储结果。